透過檔案(file)操作,將資料存入檔案,避免當發生程式結束或電腦重啟時,無法重新讀取資料。
檔案路徑
進行檔案處理前,須先了解作業系統的檔案結構和檔案系統路徑。
1.相對路徑(relative path):
相對於目前工作目錄或某個特定位置的路徑,它指定了如何到達目標文件或目錄的位置。相對路徑可能包含以下元素:
.
:代表目前資料夾位置。..
:代表上一層資料夾。/
:在某些作業系統(如 Unix-like 系統)中,代表根(root)資料夾。
相對路徑的重要特點是它的位置會相對於某個參考點而變化。例如,如果你的目前資料夾是 /home/user,則相對路徑 ./documents 可能指向 /home/user/documents。
2.絕對路徑(absolute path):
絕對路徑提供了從文件系統的根目錄到目標文件或目錄的完整路徑。它指定了目標的確切位置,不受目前工作目錄的影響。絕對路徑通常以根目錄(如/
、C:\
)為起始點。
絕對路徑的一個例子是 /home/user/documents/file.txt。在這種情況下,無論目前資料夾的位置如何,該路徑都指向相同的位置。
簡單來說,可以將左邊或右邊視為相對路徑,直接報門牌地址即為絕對位置的概念。
圖片來源:Python 程式設計入門共學營
若在bacon資料夾下的檔案要定位到fizz
資料夾下的spam.txt
,相對路徑為 .\fizz\spam.txt
,絕對路徑為:C:\bacon\fizz\spam.txt
。
fizz
資料夾在bacon
資料夾裡面和bacon
資料夾下的檔案是同一層使用 .\
。
若是要指到 eggs
資料夾下的 spam.txt
,則要先回到上一層資料夾..\
,所以相對路徑是..\eggs\spam.txt
,絕對路徑為:C:\eggs\spam.txt
。
windows用戶需要在路徑字串前加上r
,代表後方是raw string,才不會把\
視為跳脫字元。
r'C:\Program Files (x86)\Tesseract-OCR\tesseract'
若沒使用 r
則需要跳脫字元 \
來表示路徑符號 \\
:
'C:\\Program Files (x86)\\Tesseract-OCR\\tesseract'
python 檔案處理
使用open()
開啟檔案,使用close()
關閉檔案。使用open()
會建立一個file物件(object),依靠file物件來識別目前操作的檔案。
open('檔案名稱 filename', '模式 mode')
實際範例:
# 讀取模式
file_object = open('./demo.txt', 'r')
# 寫入模式
file_object = open('./demo.txt', 'w')
open()
函式中,開頭是要操作的目標檔案位置,後面參數是選擇模式(mode),而+
,代表是否支援檔案更新,代表支援讀寫功能。
mode模式 | 當檔案已經存在 | 檔案不存在 |
---|---|---|
r | 開啟唯讀檔案 | 發生錯誤 |
w | 清除檔案內容後寫入 | 建立寫入檔案 |
a | 將內容寫到檔案最後面 | 建立寫入檔案 |
r+ | 開啟讀寫檔案 | 發生錯誤 |
w+ | 清除檔案內容後讀寫入 | 建立讀寫檔案 |
a+ | 將內容讀寫到檔案最後面 | 建立讀寫檔案 |
關閉檔案
在Python中,我們使用 open('檔案路徑', '讀寫模式')
這個語句來建立一個文件物件。請記住,在使用完畢後要使用 close 關閉文件,以防止可能的記憶體流失或系統資源的浪費。
file_object = open('./demo.txt', 'r')
file_object.close()
讀取檔案
讀取檔案的方法有三個:read([size])
、readline()
和 readlines()
。
data.txt
hello
world
i
love
python
- read([size]):讀取整個檔案
檔案當前位置起讀取size大小的位元組(bytes)。若無參數 size,則表示讀取到檔案結束為止(讀取整個檔案),回傳整個檔案內容。
2.readline([size]):每次讀出一行內容#hello #world #i #love #python file_object = open('./demo.txt', 'r') # 讀取全部檔案案內容變成字串 print(file_object.read()) file_obj.close()
因此在讀取時佔用記憶體較小,比較適合大型檔案,回傳一行字串。也可選擇性傳入 size 參數
```
file_obj = open('./data.txt', 'r')
line_nmu只是一個占位符號,可以隨意命名,只要它在程式中是唯一且具名稱意義即可。
range(5)即印出第0行~第5行的內容
for line_num in range(5):
print(file_obj.readline())
file_obj.close()
3.readlines():讀取所有行並以列表形式返回
當讀取大型檔案會比較佔記憶體,不建議用於讀取大型檔案
#讀取檔案錯誤
***
若出現"no such file"代表檔案路徑有誤或是沒有該檔案
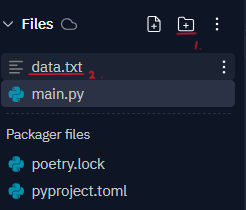
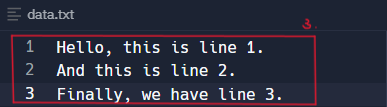
1.點選按鈕新增檔案
2.編輯檔案名稱
3.檔案內容
#python寫入檔案
***
file_object = open('./demo.txt', 'w')
若需要換行可以於字串最後加入: \n 為換行符號
file_object.write('Java is awesome\n')
file_object.write('Python is awesome')
file_object.close()
#練習
data.txt:
hello
world
hello
python
執行以下` read([size])`、`readline()` 和 `readlines()` 讀取` data.txt` 檔案方式。
1.` read([size])`
hello
world
hello
python
file_obj = open('data.txt','r')
print(file_obj.read())
file_obj.close()
2.`readline()`
hello
world
file_obj = open('data.txt','r')
只讀取到line2
for _ in range(2):
print(file_obj.readline())
file_obj.close()
3.`readlines()`
file_obj = open('data.txt','r')
file_item_list = file_obj.readlines()
for file_line_item in file_item_list:
print(file_line_item)
file_obj.close()
#寫入檔案
使用`write`將字串內容寫入檔案中。
`a:將內容新增至檔案最後行`
file_obj = open('data.txt','a')
file_obj.write('Java is awesome\n')
file_obj.write('Python is awesome\n')
file_obj.close()
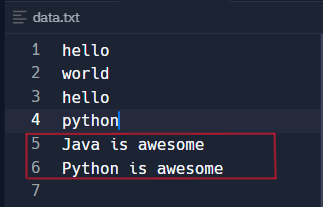
`w:清除檔案內容後寫入`
file_obj = open('data.txt','w')
file_obj.write('Java is awesome\n')
file_obj.write('Python is awesome\n')
file_obj.close()
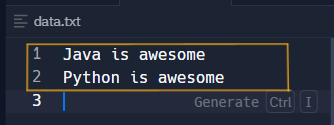
#with as 檔案操作
***
開啟檔案時(`open()`)若忘記將檔案關掉(`close()`)則會造成記憶體的浪費。然而,經常要開關非常不便,因此,為了讓開關檔案更加簡便,可以透過`with...as`,讓程式執行完後自動關閉檔案。
原data.txt內容:
Java is awesome
Python is awesome
```
with open('./data.txt','a') as file_object:
file_object.write("I love programming.")
新data.txt內容:
Java is awesome
Python is awesome
#透過a,將內容新增至檔案最後一行
I love programming.
練習
請新增一個 data.txt 檔案,裡面依序寫了 hello world1、hello world2。請使用 with ... as 方式操作 open() 和 readlines() 方法,印出每一行內容
with open('./data.txt', 'r') as file_obj:
file_item = file_obj.readlines()
for file_line in file_item:
print(file_line)